Have you ever spent hours writing code, only to have it fail in ways you never expected? Welcome to the world of bugs in coding, the unseen gremlins that haunt every developer’s work.
In this comprehensive guide, we’ll dive deep into what bugs in coding really are, explore their different types in detail, understand why they occur, and master professional techniques to squash them for good.
What Exactly is a Bug in Coding?
Imagine you're building a LEGO tower. You have instructions, but if you put the wrong piece in the wrong place, or if you skip a step, the tower might wobble, fall over, or look completely different from what you intended. In coding, a "bug" is like that wrong LEGO piece or skipped step. It's an error in the instructions (the code) that makes the computer do something it wasn't supposed to do.
So basically, a bug in coding refers to any defect, error, or flaw in a software program that causes it to produce incorrect or unexpected results—or even crash entirely.
The Origin of the Term "Bug"
The term dates back to 1947, when Harvard engineers working on the Mark II Aiken Relay Calculator found an actual moth trapped in a relay, causing a malfunction. Grace Hopper, a pioneering computer scientist, famously taped the moth into the system’s logbook with the note: "First actual case of bug being found."
Since then, the term "debugging" was coined to describe the process of finding and fixing errors in code

How Bugs Manifest in Software
Bugs can appear in various ways:
- Silent failures (code runs but produces wrong output)
- Crashes (program stops abruptly)
- Performance issues (slow execution, memory leaks)
- Security vulnerabilities (bugs hackers can exploit)
Common Types of Bugs in Coding
Not all bugs are created equal. Understanding their categories helps in diagnosing and fixing them efficiently.
1. Syntax Errors (Compile-Time Bugs)
- Definition: Violations of programming language rules (missing semicolons, unmatched brackets, typos).
- Example:
print("Hello World" # Missing closing parenthesis
- Detection: Usually caught by the compiler/interpreter before execution.
- Fix: Carefully review error messages—they often pinpoint the exact line.
2. Runtime Errors
- Definition: Errors that occur during program execution.
- Common Subtypes:
- Division by zero:
result = 10 / 0
- Null reference: Accessing an uninitialized variable.
- File not found: Trying to open a non-existent file.
- Detection: The program crashes with an exception (e.g.,
NullPointerException
in Java). - Fix: Use try-catch blocks (exception handling) to manage unexpected states.
3. Logical Errors (The Most Insidious Bugs)
- Definition: Code runs without crashing, but produces wrong results due to flawed logic.
- Example:
# Intended to calculate average, but sums instead
def average(a, b):
return a + b # Should be (a + b) / 2
- Detection: Requires thorough testing (unit tests, manual checks).
- Fix: Step-through debugging to trace where logic deviates.
4. Edge Case Bugs
- Definition: Bugs that appear only under rare or extreme conditions.
- Examples:
- A weather app failing at -40°C (Fahrenheit and Celsius intersect).
- A payment system crashing when processing $0 transactions.
- Detection: Boundary testing (testing min/max values).
- Fix: Explicitly handle edge cases in code.
Why Do Bugs Happen?
Here are some ways those "wrong LEGO pieces" get in:
- Spelling mistakes: Like writing "teh" instead of "the," computers get confused when we misspell their instructions.
- Wrong directions: If we tell the computer to add two numbers but it multiplies them instead, it's like giving it the wrong map.
- Trying to do impossible things: Like trying to put a LEGO piece where it just won't fit, a computer gets confused when we ask it to do something it can't, like dividing something by zero.
- Counting mistakes: If we tell the computer to count to ten, but it stops at nine or goes to eleven, that's a bu
Famous Historical Bugs
The Y2K Bug (Millennium Bug)
This was a major concern leading up to the year 2000. Many computer systems stored years using only two digits (e.g., "99" for 1999). People worried that when the year changed to 2000, these systems would interpret "00" as 1900, causing widespread chaos. Fortunately, extensive preparations mitigated the worst potential effects.
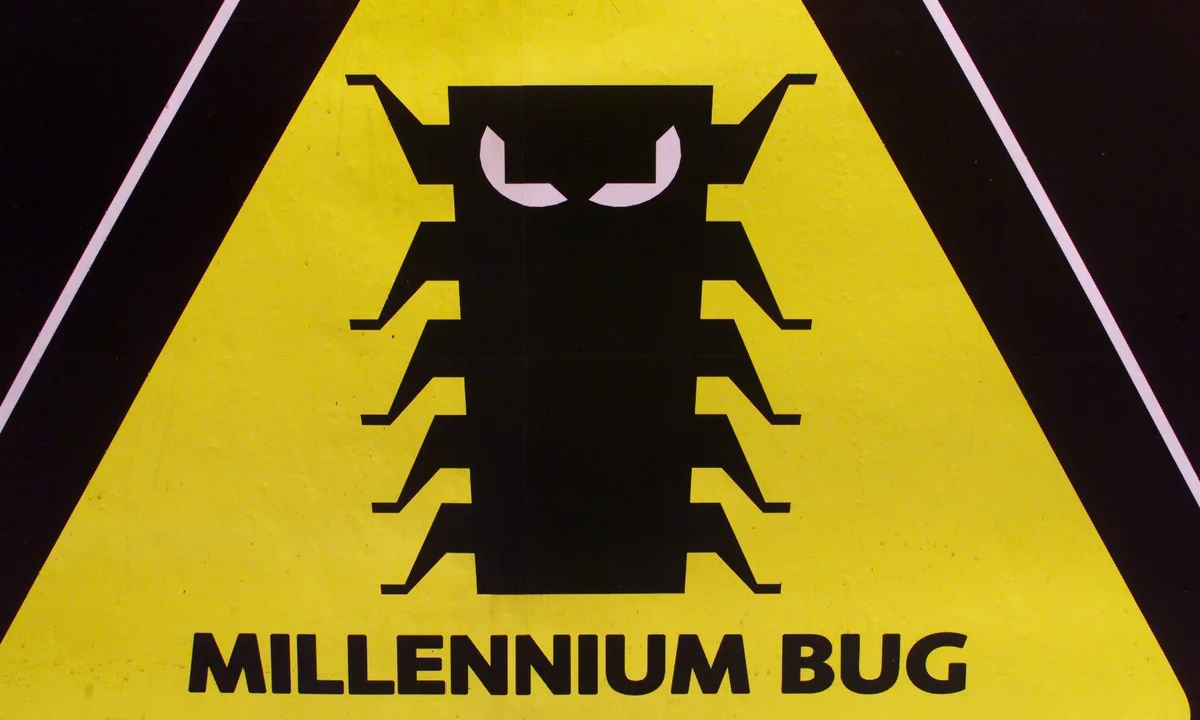
Ariane 5 Flight 501
In 1996, the maiden flight of the Ariane 5 rocket ended in disaster just 40 seconds after launch. A software error caused the rocket's navigation system to malfunction, leading to its self-destruction. The error was caused by a conversion of a 64-bit floating-point number to a 16-bit signed integer, which resulted in an overflow.
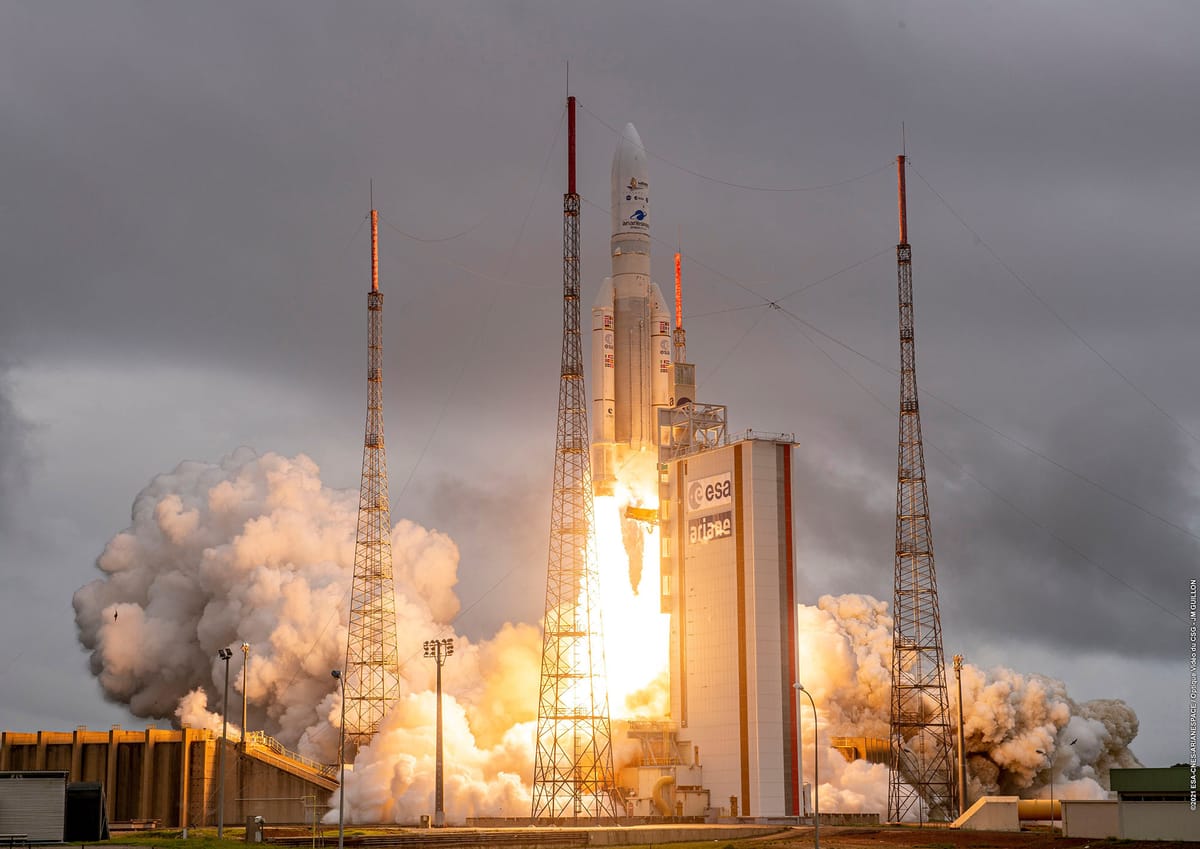
Therac-25
This radiation therapy machine was involved in several accidents in the mid-1980s, where patients received massive overdoses of radiation due to software errors. These errors highlighted the critical importance of thorough testing and safety measures in medical devices.

The Pentium FDIV bug
In 1994, Intel's Pentium processors were found to have a flaw in their floating-point division unit, resulting in inaccurate calculations. This bug caused significant public concern and financial losses for Intel.

Conclusion
Bugs are not just errors—they are inevitable milestones in every developer’s journey. From simple syntax mistakes to complex logical flaws, bugs reveal the intricacies of software development and push programmers to refine their skills.
Key Lessons About Bugs in Coding
✔ Bugs are universal – Even the most experienced developers encounter them.
✔ Debugging is a superpower – Mastering tools like breakpoints, logs, and unit tests turns frustration into solutions.
✔ Prevention beats cure – Code reviews, automated testing, and clear documentation reduce future bugs.
✔ History teaches us – The Y2K bug and Ariane 5 rocket failure prove how small oversights can have massive consequences.
Rather than fearing bugs, embrace them as learning opportunities. Each bug fixed makes you a sharper, more resilient developer.